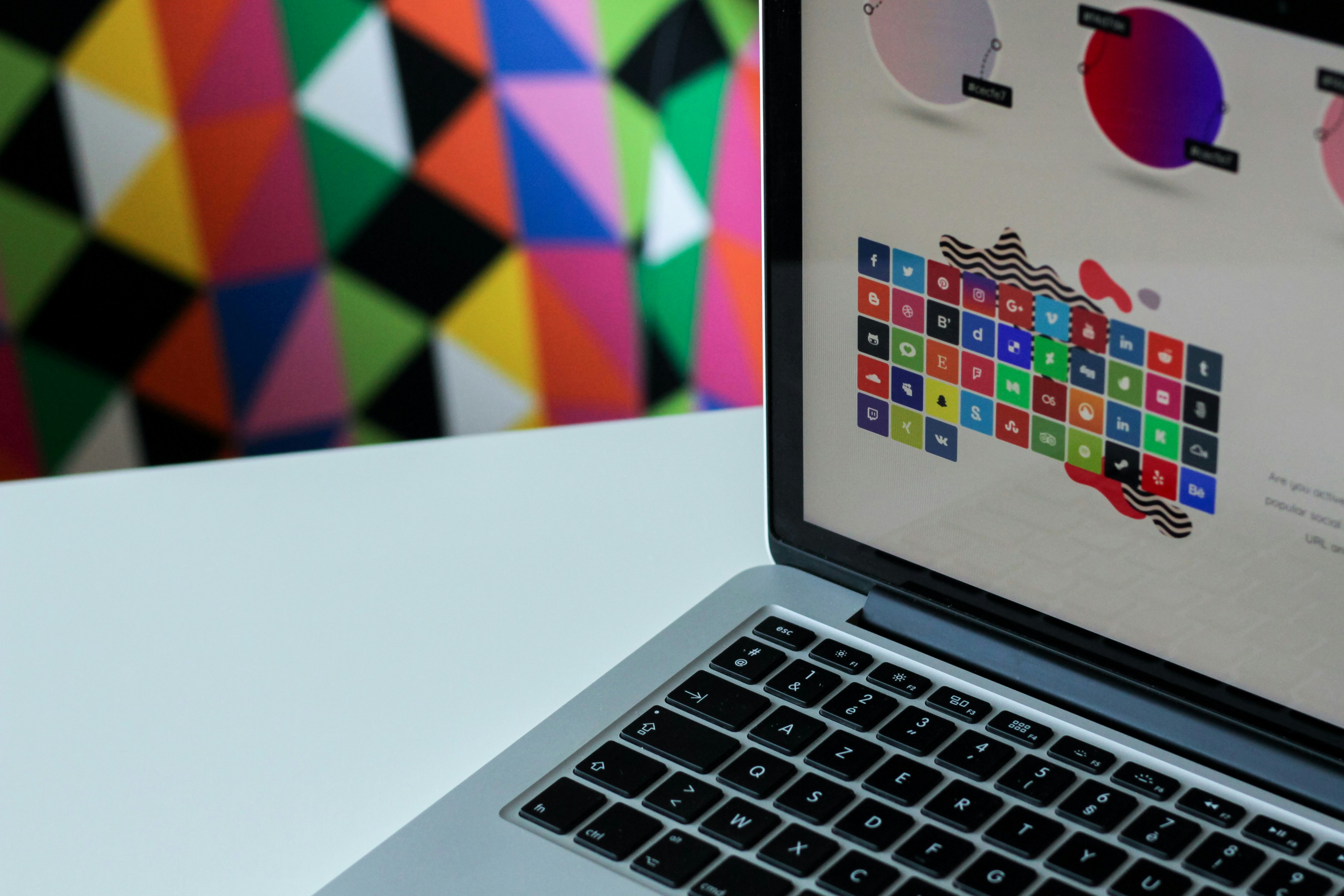
Your First Steps in Front-End Development: A Beginner's Roadmap
Description
Think front-end software development might be for you, but not sure where to start. Then this guide is for you!
Below, we'll break down everything you need to know to start your journey. You'll get an introduction to some common technologies front-end developers use daily. And don't worry if you've never written a line of code - this guide is for people with no technical background.
What is Front-End Development?
Before we begin, let's clarify what front-end software development is. A front-end software engineer works on building the parts of the web that you can see and interact with. They could work on anything from the layout of the content you see on the page to a modal that pops up when you submit a form or a sleek animation that happens when you navigate between the pages of your favorite website.
If you want a beginner-friendly guide on the differences between front-end and back-end software development, check out our other guide, What Is Software Engineering.
What is Front-End Development?
Before we begin, let's clarify what front-end software development is. A front-end software engineer works on building the parts of the web that you can see and interact with. They could work on anything from the layout of the content you see on the page to a modal that pops up when you submit a form or a sleek animation that happens when you navigate between the pages of your favorite website.
If you want a beginner-friendly guide on the differences between front-end and back-end software development, check out our other guide, What Is Software Engineering.
The Foundation: HTML & CSS
HTML: The Building Blocks of the Web
HyperText Markup Language or HTML is the structure behind every website. This structure is built with different blocks - known as elements.
HTML elements are like containers that hold different types of content. We won't go into the full dictionary of elements here, but let's look at a few. For example, imagine you're building an online version of a newspaper; here are a few of the elements you might use:
HTML: The Building Blocks of the Web
HyperText Markup Language or HTML is the structure behind every website. This structure is built with different blocks - known as elements.
HTML elements are like containers that hold different types of content. We won't go into the full dictionary of elements here, but let's look at a few. For example, imagine you're building an online version of a newspaper; here are a few of the elements you might use:
- <header> is like the masthead at the top of the page
- <nav> holds your navigation menu
- <main> contains your primary content
- <h1> to <h6> are your headlines of different sizes
- <footer> is like the publication info at the bottom
Here's a simple example of what the HTML for it might look like:
<header> <h1>The Daily News</h1> <nav> <a href="home.html">Home</a> <a href="breaking-news.html">Breaking News</a> <a href="sports.html">Sports</a> </nav> </header> <main> <h2>Top Story</h2> <p>The top story for today is ...</p> </main> <footer> <p>Copyright The Daily News</p> </footer>
Forms and Input
HTML forms are how we collect information from users. They are the digital versions of paper forms.
Here are a few of the common elements included in forms:
- <form> - The container for all form elements
- <label> - Text description for an input
- <input> - The field where users enter data
- <button> - Clickable button to submit the form
This form example collects a user's name and their email address:
<form> <label for="name">Your Name:</label> <input type="text" id="name" placeholder="John Doe"> <label for="email">Email:</label> <input type="email" id="email" placeholder="john@example.com"> <button type="submit">Send</button> </form>
Lists
Lists help keep information organized. A great use would be to display a shopping list or the steps of a recipe.
They come in two flavors: ordered and unordered. Here are some of the elements that build a list:
Lists help keep information organized. A great use would be to display a shopping list or the steps of a recipe.
They come in two flavors: ordered and unordered. Here are some of the elements that build a list:
- <ul> - Unordered list (bullet points)
- <ol> - Ordered list (numbers or letters)
- <li> - List item
Below, our "Shipping list" is unordered. While the "Recipe steps" list is ordered.
<h2>Shopping list</h2> <ul> <li>Apples</li> <li>Bananas</li> <li>Bread</li> </ul> <h2>Recipe steps</h2> <ol> <li>Preheat oven</li> <li>Mix ingredients</li> <li>Bake for 20 minutes</li> </ol>
Tables
Tables are perfect for displaying structured data in rows and columns, like the web version of an Excel spreadsheet.
The elements that make up a table:
- <table> - The container for your entire table
- <thead> - The header section
- <tbody> - The main content of your table
- <tr> - A table row
- <th> - A header cell
- <td> - A regular data cell
Here's an example table that shows a programming language and the year it was created:
<table> <thead> <tr> <th>Language</th> <th>Created</th> </tr> </thead> <tbody> <tr> <td>Python</td> <td>1991</td> </tr> <tr> <td>Ruby</td> <td>1993</td> </tr> <tr> <td>JavaScript</td> <td>1995</td> </tr> </tbody> </table>
Media Elements
Media elements allow you to embed different types of media on a website.
- <img> - For displaying images
- <video> - For video content
- <source> - Specifies multiple media resources
Here's an example of how you could display a picture of a dog above a video.
<img src="dog.jpg"> <video controls> <source src="dog-park.mp4" type="video/mp4"> </video>
Accessibility
Accessibility attributes help make your website usable for everyone. For example, let's look at the code for the image of a dog above. Someone using a screen reader wouldn't be able to tell what the image is. We can include an alt attribute to describe it.
<img src= "dog.jpg" alt= "A happy golden retriever playing with a ball">
CSS: Making Things Beautiful
Just writing plain HTML will put content on the page, but it won't look great. That's where CSS comes in.
With CSS, you can add color and design to the page or specify how you want it to look on a phone and a desktop. You can even use it to create animations!
There are many tutorials that will help you become effective in CSS, so let's explore some of the concepts.
CSS Rules Basics
- Start with a 'selector', which targets specific HTML element(s)
- We use curly braces {} to contain our style declarations
- Each declaration has a property and a value, separated by a colon :
- Each declaration ends with a semicolon ;
For example, here's a <p> element with some text. It also has a class attribute of "my-class" and an id attribute of "my-id".
<p class="my-class" id="my-id"> Hello world! </p>
Let's say we wanted to change the color of the text here to blue; we could either target the element by its tag name:
p { color: green; }
Or we could target it by its class:
.my-class { color: green; }
We can also use the ID:
.my-id { color: green; }
The Box Model
On a webpage, every element is a box, and the box has a few different layers.
Content Area:
- Where your content - text, images, etc. - lives
- The innermost part of the box
Padding:
- Space between your content and the border
- Can be set on each side - top, right, bottom, left - independently
- Transparent, shows the background color of the element
Border:
- The line around the padding and content
- Can have different styles - solid, dashed, dotted
- It can have different widths and colors
Margin:
- The space outside the border
- Useful to create gaps between elements
- Always transparent
We need to interact with these layers using CSS rules to control how they are displayed.
We'll create an HTML element with the class "my-class".
<div class="my-class"> <p>Hello world!</p> </div>
Then, to style that element, we'll create a rule that adds 20 pixels of padding, a 2-pixel solid black border, and 10 pixels of margin around the box.
.my-class { padding: 20px; /* Space inside the box */ border: 2px solid black; /* The box itself */ margin: 10px; /* Space around the box */ }
JavaScript
While there are many programming languages - like Python, Ruby, or Java - JavaScript is unique because it's the only programming language that runs in every web browser. This is why we use JavaScript for front-end development – it's built into every browser and ready to use!
JavaScript allows you to do everything from displaying a simple alert to creating an entire web framework.
Let's dip our toes into JavaScript by exploring some of its core concepts.
You can use variables to store information:
let username = "Alan Turing"; Or use control flow to make decisions: if (score > 50) { console.log("You won!"); } else { console.log("Try again!"); }
You can also use functions to create reusable code:
function sayHello(name) { return "Hello, " + name + "!"; }
DOM Manipulation
Front-end developers will often need to use JavaScript to interact with a webpage. To do that, they use the Document Object Model or DOM. Let's walk through an example where we use the DOM to reveal some text when a button is clicked.
Our HTML has two elements: a <button> and <p>. The <button> has an id attribute, and the <p> has a class and an id.
<button id="show-message-button">Show The Message</button> <p class="hidden" id="secret-message">The secret message</p>
We don't want our secret message displayed, so we also have this CSS rule `.hidden` to prevent it from being shown on the page.
.hidden { display: none; }
Then, write some JavaScript to reveal our secret message. Let's walk through how this works together.
First, we're using the DOM function document.querySelector to get the button, and then we're using the text elements.
Then, we're adding an "event listener" to our button. All this means is that now, whenever someone clicks the button, we want to trigger some code.
The code we want to run is a JavaScript function that removes the class "hidden," which will reveal the secret text.
// Getting an element let button = document.querySelector('#show-message-button'); let secretMessage = document.querySelector('#secret-message'); // Making something happen when the button is clicked button.addEventListener('click', function() { secretMessage.classList.remove("hidden") });
Frameworks
What is a JavaScript Framework?
The goal of a framework is to take care of much of the boilerplate code you need to create a web application. Allowing you to focus on what's unique about your app.
Imagine you're building a house. You could work with an architect to create a custom floor plan for your new home, which requires a lot of time and work, but you get to decide each aspect of your home.
Or you could work with a homebuilder to choose a pre-determined home design. You would have less control over the design of your house, but the process would be faster and standardized. This scenario is much like what a JavaScript web development gives you.
A framework provides you with a structured way to build websites and applications. Instead of writing everything from scratch, frameworks provide features like reusable components, routing, and performance optimizations.
Let's look at some of the popular frameworks.
React
React, created by Facebook, is currently the most popular JavaScript framework because it's powerful and flexible. React lets you create small, reusable pieces (called components) that work together to build complex applications.
Vue.js
Vue was created by Evan You in 2014. It's a flexible JavaScript framework that lets you start small and gradually use more features as you need them. It is particularly good at giving clear error messages and having excellent documentation.
Angular
Angular, developed by Google, is an excellent option for large, complex applications. What makes Angular shine is how it uses TypeScript - a more structured version of JavaScript - to help catch errors before they happen in production.
Svelte
Svelte is a relatively newer framework that was released at the end of 2016 by Rich Harris. It's different from other frameworks, like React, because it doesn't use a virtual DOM. Instead, Svelte compiles to vanilla JavaScript, which results in a super fast website. Developers love Svelte because it lets them do more with less code than other frameworks.