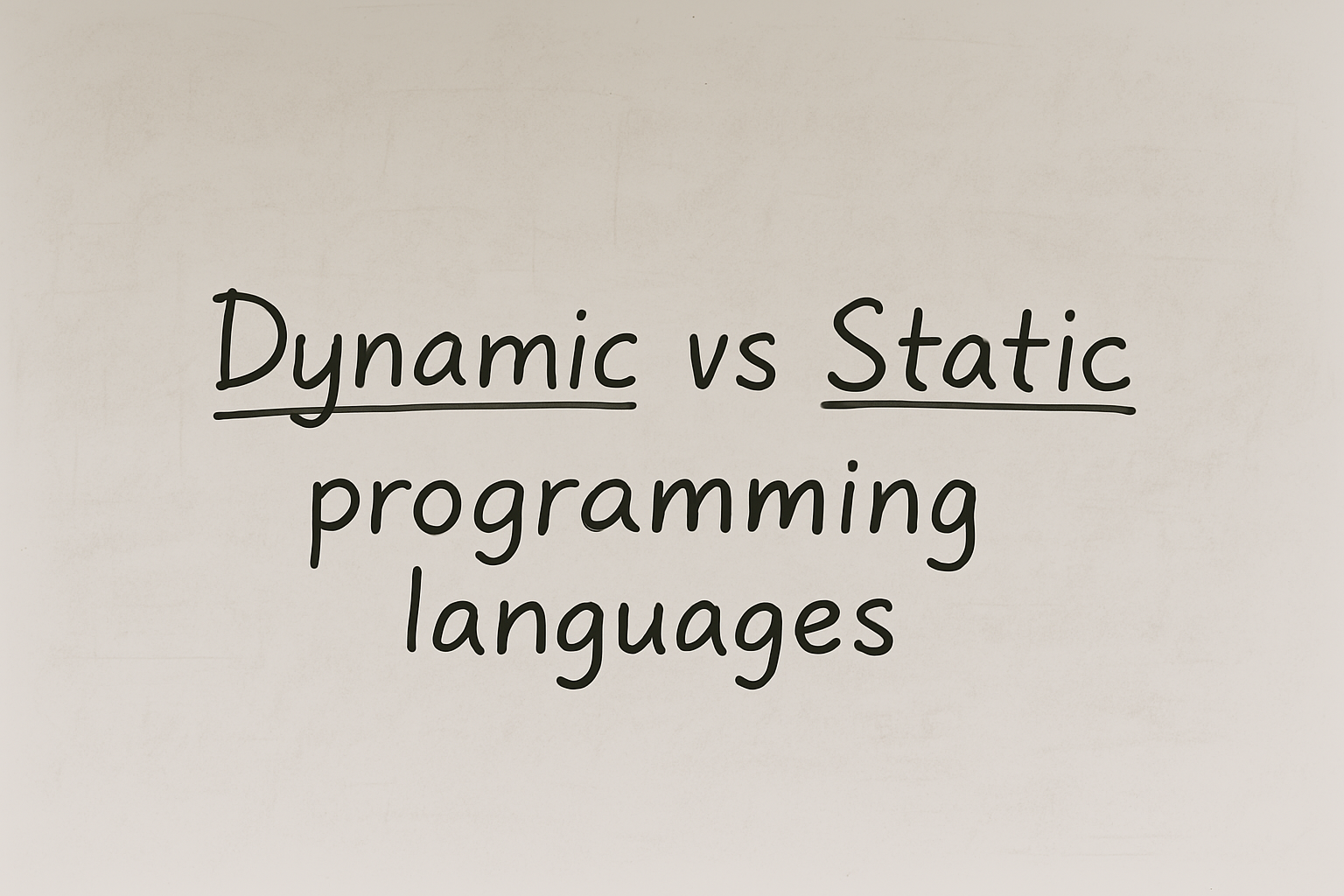
A Beginner’s Guide to Static vs. Dynamic Programming Languages
Description
Understanding the differences between language types is one of the first steps to becoming a skilled developer. In this article, we'll explore one of the most fundamental distinctions: static versus dynamic typing.
This difference affects how you write code, catch errors, and even think about programming problems.
What Are Programming Languages?
Let's step back before diving into the differences between static and dynamic languages. Programming languages are how we communicate with computers.
Suppose you think of programming languages like human languages. Just as different human languages have different grammar rules, vocabulary, and structures, programming languages have distinct rules and characteristics that make them unique.
Static vs. Dynamic: The Core Difference
The biggest difference between a static and dynamic language is how the computer program checks your program's "types" of data. So what are types?
What are "types"?
In programming, everything has a type. Numbers, text, dates, and more all have specific types. Let's look at a few examples:
- The number 42 is an integer type
- The text "Hello, World!" is a string type
- The values true or false are boolean types
Types matter because they determine the operations you can perform. For example, you can multiply two numbers, but you can't multiply a number by a text or boolean.
Static Languages: Checking Types Before Running
In a static language, the computer checks all your types before your program even runs. Think of it like having a proofreader check your essay before you submit it.
Let's see how this works with a simple example in Java (a static language):
String name = "Alice"; int age = 30; System.out.println(name + " is " + age + " years old."); // This would cause an error before running: age = "thirty"; // Error: Cannot assign a String to an int variable
The computer would catch this error during the "compilation" stage before your program runs. Because we declared age as an integer - we can't then try to assign a string -" thirty" - to it.
The Benefits of Static Languages:
Early Error Detection: Many mistakes are caught before your program runs.
Better Performance: Your code can run faster because it doesn't need to check types while running.
Tooling: Programming tools can provide more helpful suggestions since they know the types.
More Straightforward to Maintain: In larger projects, having clear types can make code easier to understand.
Static Languages:
- Java
- C++
- TypeScript
- Rust
Dynamic Languages: Checking Types While Running
With dynamic languages, type-checking happens while the program is running. Going back to our analogy, if a static language is like having a proofreader check your essay before you submit it, then a dynamic language is like having someone edit your writing as you go.
Here's an example in Ruby (a dynamic language):
name = "Bob" age = 25 puts "#{name} is #{age} years old." # Ruby won't complain about this until it runs: age = "twenty-five" # This is allowed in Ruby! # If you later tried to do: age + 10 # Only then would you get an error
In this example, our code wouldn't throw an error when we update age to be a string, but if our program later tried to add that new age ("twenty-five") to an integer, it would throw an error.
Benefits of Dynamic Languages:
Faster Development: Less time spent declaring types means you can write code more quickly
More Flexibility: Change variable types on the fly
Easier to Learn: Generally simpler syntax with fewer rules to remember
Great for Prototyping: Quickly test ideas without worrying about perfect type structures
Dynamic Languages:
- Ruby
- Python
- JavaScript
- PHP
Which Should You Learn First?
Are you new to programming? Start with a dynamic language like Ruby or Python. Here's why:
Faster Feedback Loop: You can write a little code and see results quickly.
Focus on Concepts: You can focus on learning programming concepts without getting caught up in type declarations.
Less Initial Frustration: Fewer rules mean fewer confusing error messages when starting.
However, there's real value in learning a static language, too.
Real-World Analogy
- Static languages are like carefully planning a road trip with a detailed map before leaving. You might spend more time planning, but you're less likely to get lost.
- Dynamic languages are more like taking a road trip with GPS that recalculates as you go. You can start driving sooner and figure things out along the way, but you might be more likely to get lost.
A Simple Code Comparison
Let's see a quick comparison of the same simple program in a static language - Java - and a dynamic language - Ruby:
Java (Static):
public class Greeting { public static void main(String[] args) { String name = "Alex"; int yearOfBirth = 1992; int currentYear = 2025; int age = currentYear - yearOfBirth; System.out.println("Hello, " + name + "! You are about " + age + " years old."); } }
Ruby (Dynamic):
name = "Alex" year_of_birth = 1992 current_year = 2025 age = current_year - year_of_birth puts "Hello, #{name}! You are about #{age} years old."
Notice how the Ruby code is shorter and requires less setup.
Dynamic VS Static - When to Use?
As you continue to write code, you'll discover that different languages are better suited for different things:
Static languages are good at:
- Large, complex projects with many developers
- Systems that require high-performance
- Applications where catching errors early is critical (like financial or medical software)
Dynamic languages are good at:
- Web development
- Data analysis and scientific computing
- Scripting and automation
- Rapid prototyping and startups
Suggested Learning Path
If you're just starting, here's a suggested path:
1) Begin with Ruby - a dynamically typed language - to learn core programming concepts
2) Build a few small projects to get comfortable with programming
3) Then try a static language like Java or TypeScript to understand the benefits of static typing
4) Compare how you'd solve the same problem in both types of languages
This approach gives you a well-rounded foundation and helps you understand the trade-offs between different programming approaches.
Conclusion
The "perfect" programming language is a myth. Each language has its own strengths and weaknesses and you'll often hear developers say to choose the "right tool for the job."
The static vs. dynamic distinction is just one aspect of what makes programming languages unique.
The most important thing when learning programming is getting started and building projects that interest you. As you solve problems and gain experience, practical application will make the theoretical differences between static and dynamic languages more meaningful.